Have you ever thought about how life would be without MATLAB. As it turns out there are free and open source options such as Python. We have so far restricted ourselves to MATLAB in this blog but now we venture out to find out what are the other options. Given below is a most basic Python code that calculates the Bit Error Rate of Binary Phase Shift Keying (BPSK). Compare this to our MATLAB implementation earlier [BPSK BER].
There are various IDEs available for writing your code but I have used Enthought Canopy Editor (32 bit) which is free to download and is also quite easy to use [download here]. So as it turns out that there is life beyond MATLAB. In fact there are several advantages of using Python over MATLAB which we will discuss later in another post. Lastly please note the indentation in the code below as there is no “end” statement in a “for loop” in Python.
from numpy import sqrt from numpy.random import rand, randn import matplotlib.pyplot as plt N = 5000000 EbNodB_range = range(0,11) itr = len(EbNodB_range) ber = [None]*itr for n in range (0, itr): EbNodB = EbNodB_range[n] EbNo=10.0**(EbNodB/10.0) x = 2 * (rand(N) >= 0.5) - 1 noise_std = 1/sqrt(2*EbNo) y = x + noise_std * randn(N) y_d = 2 * (y >= 0) - 1 errors = (x != y_d).sum() ber[n] = 1.0 * errors / N print "EbNodB:", EbNodB print "Error bits:", errors print "Error probability:", ber[n] plt.plot(EbNodB_range, ber, 'bo', EbNodB_range, ber, 'k') plt.axis([0, 10, 1e-6, 0.1]) plt.xscale('linear') plt.yscale('log') plt.xlabel('EbNo(dB)') plt.ylabel('BER') plt.grid(True) plt.title('BPSK Modulation') plt.show()
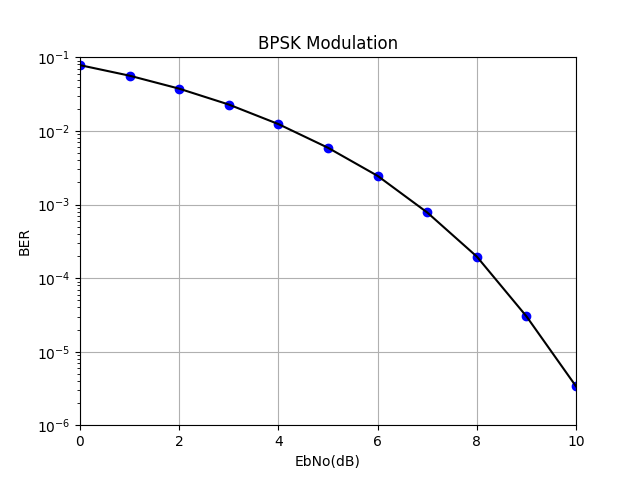
Author: Yasir Ahmed (aka John)
More than 20 years of experience in various organizations in Pakistan, the USA, and Europe. Worked as a Research Assistant within the Mobile and Portable Radio Group (MPRG) of Virginia Tech and was one of the first researchers to propose Space Time Block Codes for eight transmit antennas. The collaboration with MPRG continued even after graduating with an MSEE degree and has resulted in 12 research publications and a book on Wireless Communications. Worked for Qualcomm USA as an Engineer with the key role of performance and conformance testing of UMTS modems. Qualcomm is the inventor of CDMA technology and owns patents critical to the 4G and 5G standards.
Can you also please help with the generation of 16, 64 QAM signal in python?
Hi Abirami…that’s a good question that you have asked. Instead of giving you the Python code I will give you the algo for 16-QAM and you can try to code yourself. That will be more rewarding 🙂
1. Generate a uniform random variable (RV) between 0 and 1
2. Generate the in-phase values (-3, -1, +1, +3) according to the following look-up table
RV = 0 to 0.25, I = -3
RV = 0.25 to 0.50, I = -1
RV = 0.50 to 0.75, I = +1
RV = 0.75 to 1.00, I = +3
3. Generate another uniform random variable between 0 and 1
4. Repeat the above process for the quadrature value (Q)
5. Once I and Q are generated, I+j*Q gives you the required 16-QAM symbol
Hello,
I would like to request kindly permission using the figure “BPSK Bit Error Rate” ploted in python. the final use is for a Master Thesis in National University of Colombia.
Kindly,
Germán Chávarro
Master student
Yes please use it if its useful…that’s the whole purpose…but do not forget to reference it properly…